Prof. Vladimir A. Dobrushkin
This tutorial contains many Matlab scripts.
You, as the user, are free to use all codes for your needs, and have the right to distribute this tutorial and refer to this tutorial as long as this tutorial is accredited appropriately. Any comments and/or contributions for this tutorial are welcome; you can send your remarks to
I. The build-in matlab function ode45
matlab can be used to solve numerically second and higher order ordinary differential equations subject to some initial conditions by transfering the problem into equivalent 2 x 2 system of ordinary differential equations of first order. A function for numerical solution of such systems is, for example, \( \texttt{ode45} \) . Documentation for \( \texttt{ode45} \) states the standard usage format. One can get more advanced information by including more input/output parameters, but we start with a simplest case.
[Tout, Yout] = ode45(ODEFUN,TSPAN,Y0)
Deciphering this definition, we see that \( \texttt{ode45} \) ;
function solveDifferentialEquation
%This function solves y'+y = x from starttime to endtime with y(0) = intialCondition
%Parameter initializations
starttime = 0;
endtime = 20;
initialCondition = -2;
function yprime = SlopeFunction(x,y)
yprime = y - x;
end
[xVals,yVals] = ode45(@SlopeFunction,[starttime,endtime],initialCondition);
plot(xVals,yVals);
end %Function Complete
Example. We present four equations of first, second, and third order to demonstrate how ode function works. To solve a differential equation, the symbolic function y(t) must be defined using the syntax "syms" as such:
syms y(t)
dsolve("differential equation", "initial condition").
To express derivatives, the diff function is used. The input of the diff function is
diff("variable being differentiated", "variable that the first variable is being differentiated with respect to").
Standard boolean operators such as "==" denoting "equal to" may be used. The following line of code solves the differential equation y' = y*t^2 for y, with the initial condition y(0) = 2
y(t) = dsolve(diff(y,t) == y*t^2, y(0) == 2)
Nonlinear differential equations are differential equations where the dependent variable or any of its derivatives are not linear. They are generally more difficult to solve, but Matlab can solve these too! It is important to note that even if there are initial conditions, nonlinear differential equations can have more than one solution. matlab will list all solutions regardless. In the following example, a function x(t) is a solution of the nonlinear differential equation (x' + 2x)^2 = 3 subject to the initial conditions x(0) = 0. Notice there are two solutions.
syms x(t)
x(t) = dsolve((diff(x,t) + 2*x)^2 == 3, x(0) == 0)
x(t) =
3^(1/2)/2 - (3^(1/2)*exp(-2*t))/2
(3^(1/2)*exp(-2*t))/2 - 3^(1/2)/2
To solve a second order ODE with initial conditions, a second function must first be defined to represent the derivative of the initial function you're solving for. This function is then given a value in the initial conditions.
Additionally, to denote a second derivative, the diff function gains a third argument equaling the independent variable to show that the first argument is being differentiated again. To clarify, the first argument is differentiated with respect to all subsequent arguments to get the output.
In this example, the equation y'' = sin(3*x) - y is solved for y with theinitial conditions y(0) = 1 and y'(0) = 1. Finally, we apply simplify command to make the end result look nicer.
syms y(x)
Dy = diff(y);
y(x) = dsolve(diff(y, x, x) == sin(3*x) - y, y(0) == 1, Dy(0) == 0);
y(x) = simplify(y)
y(x) =cos(x) + sin(x)/2 - (cos(x)^2*sin(x))/2
Finally, to solve third order ODEs, the same logic is used. A third symbolic function representing the second derivative of the solution
function must be introduced to properly define the initial conditions. In our the last example, the differential equation u''' = u is solved for u with the initial conditions u(0) = 1, u'(0) = -1, and u''(0) = 0. Notice that the diff function can also be written with the dependent variable.
syms u(x)
Du = diff(u, x);
D2u = diff(u, x, 2);
u(x) = dsolve(diff(u, x, 3) == u, u(0) == 1, Du(0) == -1, D2u(0) == 0)
u(x) =
exp(-x/2)*cos((3^(1/2)*x)/2) - (3^(1/2)*exp(-x/2)*sin((3^(1/2)*x)/2))/3
Now to practice, try solving the differential equation y''*x^2 + x*y' = y with the initial conditions y(0)=0 and y'(0)=1. The answer should be y = x
Example. We consider a simple RLC circuit in series that is modelled by the differential equation
\[ \frac{{\text d}^2 i}{{\text d} t^2} + 2\alpha \, \frac{{\text d} i}{{\text d} t} + \omega^2 i(t) =0, \] where \( i(t) \) represents the current traveling through the circuit as a function of time, t. The coefficients \( \alpha \) and \( \omega^2 \) incorporate the constants used in a resistor, inductior, and a capacitor. Additionally, \( \alpha \) and \( \omega^2 \) represent two distinct types of angular frequency, the fotmer is known as the neper frequency and the latter is known as the angular resonance frequency. In other words, a higher \( \alpha \) value will increase resistance in the circuit and a smaller value of \( \alpha \) will have the opposite effect on the circuit. Therefore, the harmonic oscillations of the current through the circuit decay with increase values of \( \alpha \) and approach zero.
First we define simplecircuit function
function simplecircuit
%initial conditions
omega=1;
a=.4; %set a to be whatever damping factor you wish to observe graphically
[t,y] = ode45(@circuit,[0 16], [-1 0]);
plot(t,y(:,2));
xlabel('time');
function dy = circuit(t,y)
dy = zeros(2,1);
dy(1) = y(2);
dy(2)=-(2*a*y(2))-(omega*omega*y(1));
end
end
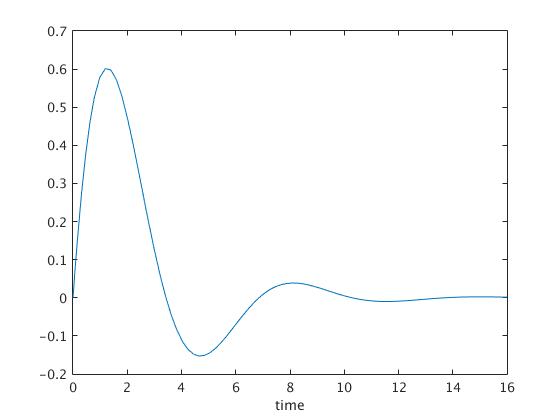
function circuitequation
%initial conditions are taken -1, and 0 (for simplicity)
[t_1,y_1] = ode45(@circuit,[0 16], [-1 0]);
plot(t_1,y_1(:,2),'b');hold on
hold off
xlabel('time');
ylabel('Current, I');
function dy = circuit(t,y)
dy = zeros(2,1);
dy(1) = y(2);
dy(2)=-(2*.4*y(2))-(1*1*y(1));
%here a is set to .4 but can be set to any value as long as that value corresponds to the same value for a in the initial conditions section of simplecircuit.m
end
end
Let us see how we can solve a first order ODE subject to an initial condition. In general, it will be in the form:
Example. Let us solve the initial value problem
The following matlab code numerically solves the given initial value problem with \( \texttt{ode45} \)
and plot it on the same axis along with the exact solution.
close all
% define anonymous function/slope f(t,y)
f = @(t,y) 6*t -3*y +5;
% call ode45
[t,y] = ode45(f, [0,1],3);
% plot numerical solution and exact solution
plot(t, y, 'b-', t, 2*exp(-3*t)+2*t+1, 'r:');
legend('numerical', 'exact', 'Location', 'best');
legend boxoff
shg